AWS Bedrock Agents empower organizations to build intelligent, generative AI applications that execute complex, multistep workflows across various systems. One of the fundamental building blocks of these agents is the action group. In this post, we’ll explore in depth what an action group is, how it functions within the agent ecosystem, and touch on related concepts—complete with practical code examples.
What Is an Action Group?
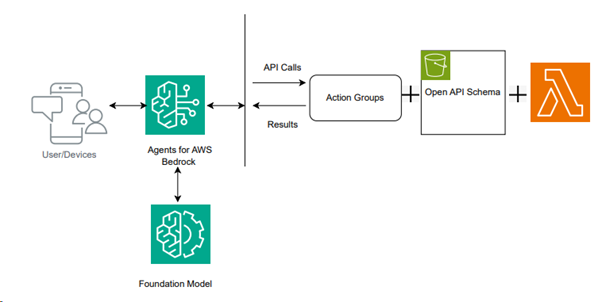
An action group in AWS Bedrock Agents is a configurable collection of functions that the agent can invoke during its orchestration process. It bridges the high-level reasoning of a language model with concrete actions like data retrieval or external API calls. In simpler terms, action groups are the means by which an agent executes specific tasks, such as fetching customer settings or verifying login statuses.
Key Characteristics:
- Function Definitions: Each action group lists one or more functions with defined parameters. These functions encapsulate discrete tasks.
- Simplified Configuration: Developers only need to specify basic details (e.g., function name, description, and required parameters), minimizing the complexity of integration.
- Integration Flexibility: Action groups can either trigger AWS Lambda functions or use the “return of control” mechanism—allowing the calling application to handle the action externally.
A simple JSON snippet that represents an action group configuration might look like this:
jsonCopyEdit{
"actionGroupName": "retrieve-customer-settings",
"description": "Retrieve customer settings using customer email",
"functions": [
{
"name": "retrieve-customer-settings-from-crm",
"description": "Fetches customer settings from the CRM using the provided email",
"parameters": [
{
"name": "email",
"type": "string",
"description": "Customer email address",
"required": true
}
]
}
]
}
How Do Action Groups Work?
During the orchestration process, the agent uses chain-of-thought reasoning to decompose a high-level request into actionable steps. When it determines that an external action is required, the agent triggers an action group. Here’s what happens next:
- Parameter Extraction: The agent parses the user input to extract relevant information. For instance, it might extract an email address from a customer support query.
- Function Invocation: Based on the action group’s configuration, the agent calls the associated function—either by invoking an AWS Lambda function or by returning control to the calling application.
- Response Processing: The output from the function is then fed back into the agent’s workflow to continue the conversation or complete a multistep task.
Example: AWS Lambda Function for an Action Group
Below is a sample Python code snippet that illustrates how a Lambda function might handle an action group invocation. This function processes two hypothetical action groups: one for retrieving customer settings and another for checking login status.
pythonCopyEditimport json
def lambda_handler(event, context):
# Extract key details from the incoming event
agent = event.get("agent")
action_group = event.get("actionGroup")
function_name = event.get("function")
parameters = event.get("parameters", [])
# Determine which function to call based on the action group and function name
if action_group == "retrieve-customer-settings":
if function_name == "retrieve-customer-settings-from-crm":
# Extract the 'email' parameter from the parameters list
email = next((p['value'] for p in parameters if p['name'] == 'email'), None)
response_data = retrieve_customer_settings(email)
elif action_group == "check-login-status":
if function_name == "check-customer-login-status":
# Extract the 'customer_id' parameter from the parameters list
customer_id = next((p['value'] for p in parameters if p['name'] == 'customer_id'), None)
response_data = check_login_status(customer_id)
else:
response_data = {"error": "Invalid action group or function."}
# Format the response as expected by AWS Bedrock Agents
response_body = {"TEXT": {"body": json.dumps(response_data)}}
return {
"response": {
"actionGroup": action_group,
"function": function_name,
"functionResponse": {"responseBody": response_body}
},
"messageVersion": event.get("messageVersion")
}
def retrieve_customer_settings(email):
# Simulate a CRM lookup (replace with actual integration code)
return {"customer_id": "12345", "settings": {"language": "en", "timezone": "UTC"}}
def check_login_status(customer_id):
# Simulate checking a login system (replace with actual integration code)
if customer_id == "12345":
return {
"status": "not verified",
"reason": "Email not verified",
"solution": "Please verify your email address"
}
else:
return {"status": "verified"}
Return of Control: Enhancing Flexibility
An innovative aspect of AWS Bedrock Agents is the ability to return control. Instead of directly invoking a Lambda function for every action, an agent can return control back to the calling application. This is especially useful when:
- Integrating with external systems: The calling application might already have logic to handle API calls.
- Handling long-running tasks: Processes that exceed the 15-minute AWS Lambda timeout can be managed externally, while the agent’s orchestration flow continues seamlessly.
- Optimizing resource use: Asynchronous processing ensures that the overall system remains responsive and efficient.
Additional Related Concepts
Action groups work in concert with several other key features in AWS Bedrock Agents:
- Chain-of-Thought Reasoning: This enables the agent to logically break down a user’s query into multiple, actionable steps.
- Knowledge Base Integration: Agents can pull verified information from internal knowledge bases to ensure responses are data-driven and accurate.
- Prompt Engineering: Clear and concise instructions help guide the agent on when to trigger specific action groups, ensuring consistency and reliability.
Benefits of Using Action Groups
Incorporating action groups into your AWS Bedrock Agent workflows offers several advantages:
- Modularity: Developers can build and maintain small, focused functions that perform specific tasks, then integrate them into larger systems.
- Speed to Market: Simplified configuration and flexible integration options accelerate the development and deployment of AI-driven applications.
- Enhanced Accuracy: Direct integration with data sources ensures that responses are both accurate and contextually relevant.
- Cost Efficiency: Leveraging AWS’s scalable infrastructure means you only pay for what you use—especially when long-running processes are handled outside of Lambda.
Conclusion
AWS Bedrock Agents are transforming how organizations build intelligent applications by converting complex requests into a series of discrete actions through action groups. These groups not only simplify the integration of external systems and APIs but also provide the flexibility needed for asynchronous processing via return of control. By combining action groups with chain-of-thought reasoning, knowledge base integration, and clear prompt engineering, AWS Bedrock Agents empower developers to create scalable, accurate, and cost-effective AI solutions.
Explore AWS Bedrock Agents today and see how action groups can be the key to unlocking efficient AI automation in your workflows.
Also Read: LangGraph: An Introduction to the LangGraph Components